|
Getting Started with Ch Mechanism Toolkit
To help you
to get familiar with
Ch Mechanism Toolkit,
a sample program will be used to illustrate
basic features and applications of Ch Mechanism Toolkit.
Problem Statement:
Link lengths of a fourbar linkage shown below
are given as follows:
r1 = 12 cm, r2 = 4 cm, r3 = 10 cm,
r4 = 7 cm.
The phase angle for the ground link is theta_1 = 0,
the coupler point P is defined by the distance
rp = 5 cm and constant angle beta = 20 degree.
Plot a branch of coupler curves for the coupler point
and animate the fourbar linkage.
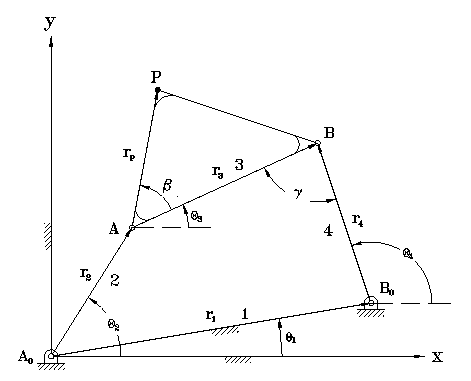
This is a crank-rocker four-bar linkage.
The code listed below is a Ch program
using Ch Mechanism Toolkit for solving this problem.
This program is shiped with Ch Mechanism Tolkit.
It is located in
CHHOME/toolkit/demos/mechanism/chapters/startup/animationcr.ch
where
CHHOME is the Ch home directory such as
C:/ch.
#include
int main() {
/* specify a crank-rocker four-bar linkage */
double r1 = 0.12, r2 = 0.04, r3 = 0.10, r4= 0.07;
double theta1 = 0;
double rp = 0.05, beta = 20*M_PI/180;
int branchnum = 1;
class CPlot plot;
class CFourbar fourbar;
fourbar.setLinks(r1, r2, r3, r4, theta1);
fourbar.setCouplerPoint(rp, beta);
fourbar.plotCouplerCurve(&plot,branchnum);
fourbar.animation(branchnum);
return 0;
}
The first line of the program
#include
includes the header file fourbar.h
which defines the class CFourbar, macros, and prototypes
of member functions. Like a C/C++ program, a Ch program
will start to execute at the main() function
after the program is parsed. The next three lines
double r1 = 0.12, r2 = 0.04, r3 = 0.10, r4= 0.07;
double theta1 = 0;
double rp = 0.05, beta = 20*M_PI/180;
define the four-bar linkage and coupler point.
Note that the link lengths are specified in meters.
The macro M_PI for pi is defined in the header file
math.h which is included in the header file
fourbar.h.
For a crank-rocker four-bar linkage,
there are two circuits or branches. The branch number
is selected in the program by integer variable
branchnum. Line
class CPlot plot;
defines a class CPlot for creating and
manipulating two and three dimensional plotting.
The CPlot class is defined in header file chplot.h
which is included in fourbar.h header file.
Line
class CFourbar fourbar;
constructs an object of four-bar linkage.
Line
fourbar.setLinks(r1, r2, r3, r4, theta1);
fourbar.setCouplerPoint(rp, beta);
specify the demensions of the four-bar linkage.
The member function setLinks()
has five arguments. The first four arguments specify the
link lengths and the fifth one is the phase angle for
link 1.
The member function setCouplerPoint()
specifies a coupler point with
two arguments, the first one for distance and the second one for
the phase angle as shown in the above Figure.
Like C++, the keyword class is optional in Ch.
Line
fourbar.plotCouplerCurve(&plot,branchnum);
computes and plots the coupler curve for the branch
specified in the second argument.
Member function plotCouplerCurve() has two arguments.
The first argument is a pointer to an existing object
of class CPlot. The second argument is the branch number
of the linkage.
The coupler curve, when
the above program is executed, is shown in the figure below.
Line
fourbar.animation(branchnum);
creates an animation of the four-bar linkage for the branch
specified in its argument.
The animation is shown below
when the program is executed.
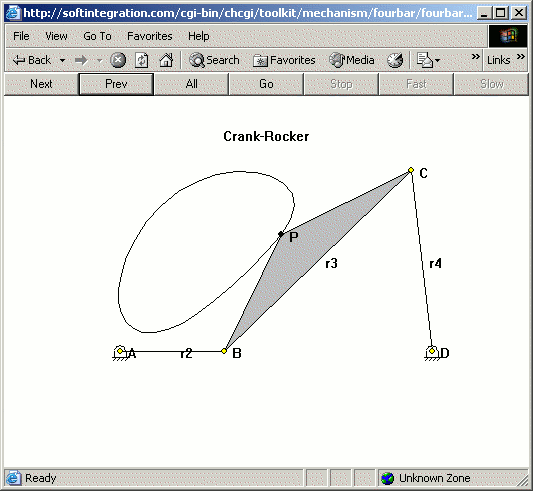
|